How best to structure a large script project?
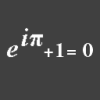
Hi,
To make development of my large project manageable, I've split it into a number of separate files, which I "include()" in my main .dsa file. (I'm not concerned about any multi-file distribution issues at present).
The documentation for Global.include() says:
"This function should only be called within the global scope of the script; it should not be called within a nested scope and it should not be called inline."
So all the include() commands are issued in the outermost scope of the script, before the main function of the script, within which I assume would be "nested scope".
It all appears to work OK so far, but I'm concerned about how much "pollution" of the Global namespace this causes, and how much is tolerable:
If an object "class" is declared in the global scope of the script (as it would be when include()ed from a separate file), does that count as only 1 global symbol, or do each of its member functions count as global symbols? (I assume the member variables don't?).
To illustrate, is the following an OK way to do things?:
A separate file that will be included:
// MyTypeDefA.dsa// Constructor:MyTypeDefA.superclass = Object;function MyTypeDefA(){ // Member variables: this.m_nVar001 = 1; this.m_nVar002 = 2; ...etc. this.m_nVar999 = 999;};// Member functions:MyTypeDefA.prototype.function001 = function() { ...etc. };MyTypeDefA.prototype.function002 = function() { ...etc. };...etc.MyTypeDefA.prototype.function999 = function() { ...etc. };
Another separate file that will be included:
// MyTypeDefB.dsa// Constructor:MyTypeDefB.superclass = MyTypeDefA;function MyTypeDefB(){ // Member variables: this.m_sVar001 = " 1"; this.m_sVar002 = " 2"; ...etc. this.m_sVar999 = "999";};// Member functions:MyTypeDefB.prototype.process001 = function() { ...etc. };MyTypeDefB.prototype.process002 = function() { ...etc. };...etc.MyTypeDefB.prototype.process999 = function() { ...etc. };
The main script file:
include( "MyTypeDefA.dsa" );include( "MyTypeDefB.dsa" );// Use an anonymous function as this script's Main Routine:( function() { // Create some instances: var g_oInstanceA001 = new MyTypeDefA(); var g_oInstanceA002 = new MyTypeDefA(); ...etc. var g_oInstanceA999 = new MyTypeDefA(); var g_oInstanceB001 = new MyTypeDefB(); var g_oInstanceB002 = new MyTypeDefB(); ...etc. var g_oInstanceB999 = new MyTypeDefB(); ...etc.: do stuff... // Exit.// Finalize the anonymous function, and immediately invoke it as this script's Main Routine:} ) ();
... or is there a better way in DAZ Script?
Thanks for any feedback.
Comments
The usage displayed in the example above is fine. Moving the
include()
statements into the anonymous function would also be fine. What the documentation is intending to discourage is usage within a scope that does not serve as the main loop; i.e., cases that increase the likelihood of failed attempts at including the same file more than once (see the comments in the documentation regarding the prevention of circular references). You can think ofinclude()
as a very simple form of theimport
statement that has been formalized in ECMA-262 6th edition, as that is the intent;import
has been a (future) reserved word since ECMA-262 1st edition, but only within the last year became standardized. As Daz Studio 4.9.x is built on Qt 4.8.7 (DS 4.5.0.114 was built on Qt 4.8.1, DS 4.0.2.55 was built on Qt 4.7.0), and thus the ECMA-262 edition 5.1 standard, we don't have support for theimport
statement (yet), so we provideinclude()
to bridge the gap until we do.Under the hood what is happening is, upon script execution and immediately prior to evaluation, the contents of the referenced script is expanded in place, replacing the statement as if said contents lived in the script at that position. This is only true, however, for the first occurrence of the statement for a given (canonical) path.
-Rob
Rob, thank you for the comprehensive reply.