Absence of boolean variabl vs FALSE value of variable
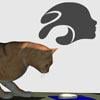
I've been writing a script to read certain values from a .dsf morph asset file. One of the things I want to check is whether the morph is hidden. Unfortunately sometimes the visible variable is missing from the asset file, and DS treats that as being visible = true, but in scripting using
if ( ! ourObject.modifier_library[ n ].channel.visible )
is true both if the variable is present and set to false (morph hidden) and if the variable is absent (morph visible). I've got around this by sending ourObject.modifier_library[ n ].channel to a custom checker function:
function morphVis ( object ) {
// find if object contaisn a property "Visible"
// if it does, return its value, if not return true
// as lack of the property seems to be treated that way
var str;
for ( var n in object ) {
str = "" + n;
if ( str == "visible" ) {
return n.value;
}
}
return true;
}
and it occurs to me I could also use JSON.stringify( ourObject.modifier_library[ n ].channel ).find( "visible" ) as a test, but both seem somewhat Heath-Robinsonish. Is there a better way to distinguish between the absence of a boolean variable in a data structure and its presence with a value of false?
Comments
something like this should work:
Ah, so I don't have to convert to string with the right approach. I'd tried n (as inb the loop ) == "visible" but that didn't work (not surprisingly), hence assigning it with the addition of "" to force it to act as a string. Thanks.
You can use Object.hasOwnProperty() to check whether an Object instance has a property. Notice that the majority of the example below is for testing/debugging.
The function above answers the question in the subject... the absence of a Boolean property vs. the value of a Boolean property.
-Rob
Thanks, that looks as if it should be simple to generalise too.